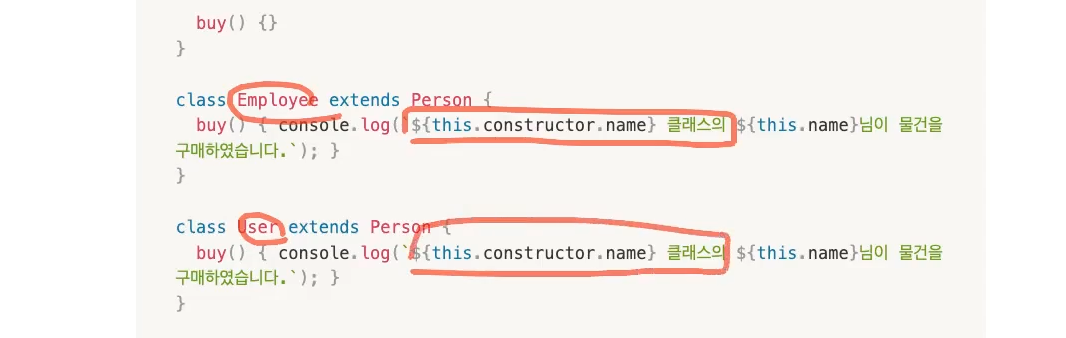
노드 심화를 배우는데 클래스 다형성 부분의 예시 보고, 저걸로 유효성 검사를 하면 괜찮을듯하다.
- joi로 먼저 유효성 검사를 하고, joi로 처리하기 어려운걸 class로 유효성 검사하기
-예시내용-
유효성 검사를 클래스 기반으로 나누는 것은 좋은 설계 패턴 중 하나입니다. 특히, 복잡한 유효성 검사를 필요로 하는 경우 객체지향 프로그래밍의 장점을 활용할 수 있습니다. 이를 통해 코드의 재사용성과 유지보수성을 높일 수 있습니다. 다음은 클래스를 사용하여 유효성 검사를 구현하는 방법에 대한 개요입니다.
### 이유 및 장점
1. **재사용성**: 유효성 검사를 여러 곳에서 재사용할 수 있습니다.
2. **유지보수성**: 유효성 검사 로직이 하나의 클래스로 캡슐화되어 있어 유지보수가 용이합니다.
3. **확장성**: 새로운 유효성 검사가 필요할 때 쉽게 클래스를 확장할 수 있습니다.
4. **테스트 용이성**: 유효성 검사를 개별적으로 테스트할 수 있습니다.
### 예제 코드
다음은 자바스크립트에서 클래스를 사용하여 유효성 검사를 구현하는 간단한 예제입니다.
#### 유효성 검사 클래스
```javascript
class Validator {
constructor() {
this.errors = [];
}
isRequired(value, message) {
if (!value || value.trim().length === 0) {
this.errors.push(message);
}
}
isEmail(value, message) {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(value)) {
this.errors.push(message);
}
}
isLength(value, min, max, message) {
if (value.length < min || value.length > max) {
this.errors.push(message);
}
}
getErrors() {
return this.errors;
}
clearErrors() {
this.errors = [];
}
}
```
#### 사용 예시
```javascript
const validator = new Validator();
validator.isRequired('John Doe', 'Name is required');
validator.isEmail('john.doe@example.com', 'Invalid email');
validator.isLength('password123', 6, 20, 'Password must be between 6 and 20 characters');
if (validator.getErrors().length > 0) {
console.log('Validation errors:', validator.getErrors());
} else {
console.log('Validation passed');
}
```
### 설명
1. **Validator 클래스**: 유효성 검사를 위한 메서드와 에러를 저장하는 배열을 포함합니다.
2. **isRequired 메서드**: 값이 존재하는지 확인합니다.
3. **isEmail 메서드**: 값이 이메일 형식인지 확인합니다.
4. **isLength 메서드**: 문자열의 길이가 지정된 범위 내에 있는지 확인합니다.
5. **getErrors 메서드**: 현재까지 수집된 모든 에러를 반환합니다.
6. **clearErrors 메서드**: 에러 배열을 초기화합니다.
### 결론
클래스를 사용하여 유효성 검사를 구현하면 코드의 재사용성과 유지보수성을 크게 향상시킬 수 있습니다. 또한, 개별적인 유효성 검사를 독립적으로 관리하고 테스트할 수 있어 개발 과정에서 발생할 수 있는 오류를 줄일 수 있습니다. 이런 방식은 특히 규모가 큰 프로젝트나 복잡한 검증 로직이 필요한 경우 매우 유용합니다.
-미들웨어로 만드는거 예시-
class CustomValidator {
constructor() {
this.errors = [];
}
isCustomValid(value, message) {
if (!/^[a-zA-Z]+$/.test(value)) {
this.errors.push(message);
}
}
getErrors() {
return this.errors;
}
clearErrors() {
this.errors = [];
}
}
const customValidationMiddleware = (req, res, next) => {
const customValidator = new CustomValidator();
customValidator.isCustomValid(req.body.name, 'Name should only contain alphabets');
if (customValidator.getErrors().length > 0) {
return res.status(400).json({ errors: customValidator.getErrors() });
}
next();
};
module.exports = customValidationMiddleware;
유저정보 받아 올 때나 게시글 등등 내용 받아올 때도 골라서 받아올 듯 해서 예시 적어둠
유저 정보를 받아오고 관리하는 로직을 클래스로 구현하는 것은 좋은 설계 패턴입니다. 이를 통해 유저 데이터를 깔끔하게 관리하고, 필요한 데이터를 선택적으로 받아올 수 있습니다. 이러한 접근 방식은 특히 객체 지향 프로그래밍(OOP)의 장점을 활용할 수 있는 좋은 방법입니다.
### 1. 유저 정보 관리 클래스 정의
다음은 유저 정보를 관리하는 클래스를 정의하는 예제입니다.
```javascript
class User {
constructor(userData) {
this.id = userData.id;
this.name = userData.name;
this.email = userData.email;
this.role = userData.role;
// 추가적인 유저 정보
}
// 유저 정보의 특정 필드만 선택적으로 반환
getUserInfo(fields) {
const info = {};
fields.forEach(field => {
if (this[field] !== undefined) {
info[field] = this[field];
}
});
return info;
}
}
```
### 2. 유저 정보 관리 클래스 사용 예제
다음은 유저 정보를 선택적으로 받아오는 예제입니다.
```javascript
// 유저 데이터 (예: 데이터베이스에서 가져온 데이터)
const userData = {
id: 1,
name: 'John Doe',
email: 'john.doe@example.com',
role: 'admin'
// 추가적인 유저 데이터
};
// 유저 클래스 인스턴스 생성
const user = new User(userData);
// 필요한 유저 정보만 받아오기
const selectedFields = ['id', 'name'];
const userInfo = user.getUserInfo(selectedFields);
console.log(userInfo); // { id: 1, name: 'John Doe' }
```
### 3. Express와 통합하여 미들웨어로 사용하기
유저 정보를 미들웨어로 통합하여 요청 시 필요한 정보를 선택적으로 받아올 수 있습니다.
```javascript
const express = require('express');
const app = express();
class User {
constructor(userData) {
this.id = userData.id;
this.name = userData.name;
this.email = userData.email;
this.role = userData.role;
// 추가적인 유저 정보
}
getUserInfo(fields) {
const info = {};
fields.forEach(field => {
if (this[field] !== undefined) {
info[field] = this[field];
}
});
return info;
}
}
// 미들웨어로 유저 정보 처리
const userMiddleware = (req, res, next) => {
// 여기서 유저 데이터를 가져옵니다 (예: 데이터베이스에서)
const userData = {
id: 1,
name: 'John Doe',
email: 'john.doe@example.com',
role: 'admin'
// 추가적인 유저 데이터
};
const user = new User(userData);
req.user = user;
next();
};
app.use(userMiddleware);
app.get('/user', (req, res) => {
const selectedFields = req.query.fields ? req.query.fields.split(',') : ['id', 'name', 'email'];
const userInfo = req.user.getUserInfo(selectedFields);
res.json(userInfo);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
### 설명
1. **User 클래스**: 유저 정보를 관리하며, `getUserInfo` 메서드를 통해 필요한 필드만 반환합니다.
2. **User 인스턴스 생성**: 유저 데이터를 받아 `User` 클래스의 인스턴스를 생성합니다.
3. **User 정보 선택적으로 반환**: `getUserInfo` 메서드를 통해 필요한 필드만 선택적으로 반환합니다.
4. **Express와 통합**: 미들웨어로 유저 정보를 처리하고, 요청에 따라 필요한 유저 정보를 선택적으로 반환합니다.
이러한 접근 방식을 통해 유저 정보를 체계적으로 관리하고, 필요한 데이터를 선택적으로 반환하는 기능을 구현할 수 있습니다. 이를 통해 코드의 가독성과 유지보수성을 높일 수 있습니다.
-유효성은 비즈니스 로직 이전에 먼저 실행하기-
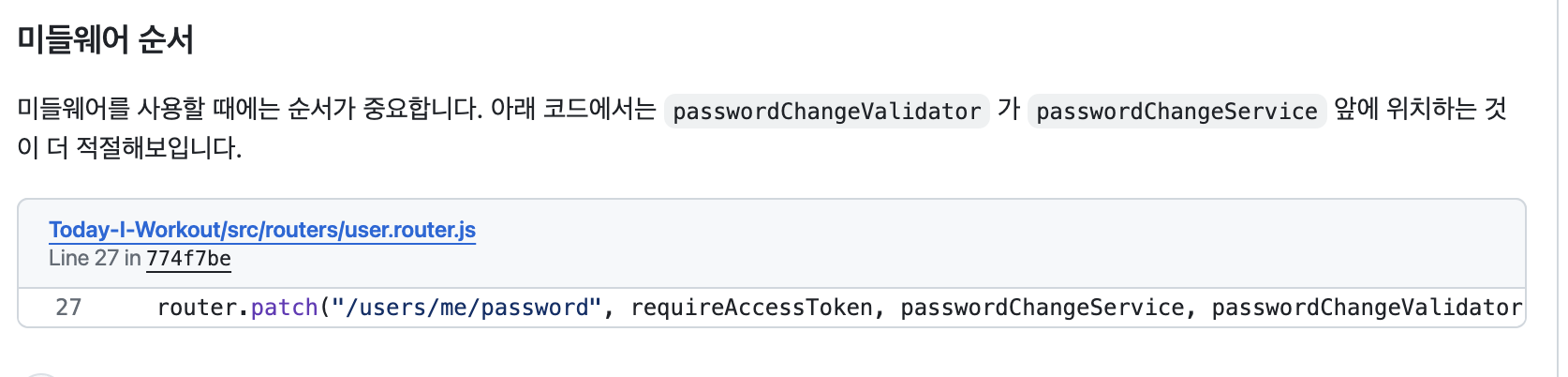
-토큰을 안 받아와서 값이 안뜨는걸 보고 순서가 중요한건 알았는데, 뒤에 두게는 로직부터 처리하고 유효성검사를 하나 했는데
완전 반대였다....기...억-
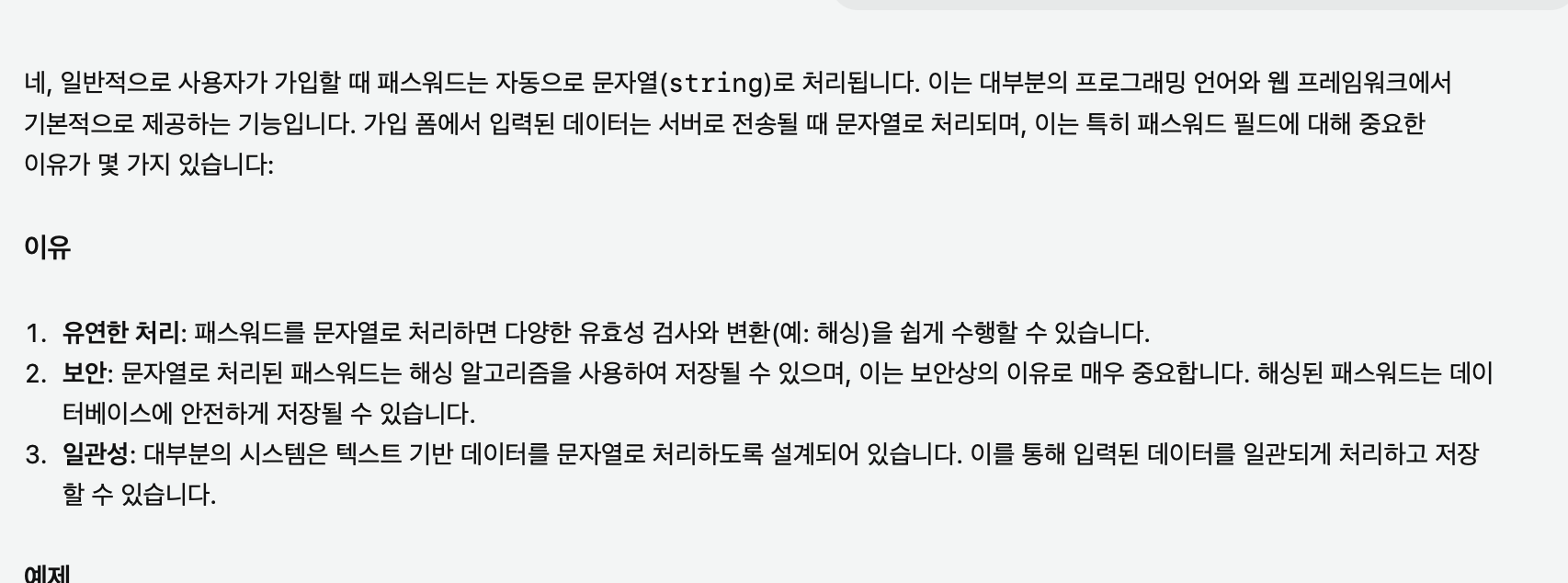
-궁금해서 찾은거-
https://momomooo.tistory.com/201
폴더 종류
개발 프로젝트의 폴더 구조는 프로젝트의 종류와 요구사항에 따라 다를 수 있지만, 일반적으로 사용되는 폴더와 그 설명을 더 추가하겠습니다.### 주요 폴더 및 설명1. **src (Sourc
momomooo.tistory.com
( 오늘 할 일 & 한 일 )
⭕️1. TIL쓰기
⭕️2. 알고리즘 문제풀기
🔺3. 노드 심화 강의 완강 >> 반이상 완료
'TIL, WIL' 카테고리의 다른 글
TIL 24.06.13 -과제, 특강(3계층 아키텍쳐 나눌때 기억할 것) (0) | 2024.06.14 |
---|---|
TIL 24.06.11 - 노드 숙련(완), 개인과제, 해설 정리, (의존성 주입의 이점) (0) | 2024.06.12 |
TIL 24.06.07 - 팀회고, 팀발표, 유튭강의 정리.... (0) | 2024.06.08 |
TIL 24.06.05 - 팀과제 프론트 (로그인 안했을때 게시글 글 쓰기 막는게 백앤드가 필요한 이유) (0) | 2024.06.06 |
TIL 24.06.04 - 팀과제 (0) | 2024.06.04 |